If you are working on a React function component, you may need to add an event to Click (or other events). Before start this article i will suggest you to read Event Handling in React Element.
You usually do:
2 3 4 |
<button onClick={handleClick}>Delete</button> |
But what if you’re going to have to pass a parameter? Say you have a list of ids, and you want to remove one by clicking on the “Delete” next to it.
You can’t do:
2 3 4 |
<button onClick={handleClick(id)}>Delete</button> |
Because the expression inside onClick will be performed on the button. This will delete all the ids in the list as soon as the app starts. This is what you need to do:
Using Arrow Function:
2 3 4 |
<button onClick={(e)=>this.handleClick(id,e)}>Delete</button> |
Using Bind Method:
2 3 4 |
<button onClick={this.handleClick.bind(this,id)}>Delete</button> |
Note:-
- In both cases, the e argument representing the react event will be passed as a second argument after the ID.
- With an arrow function, we have to pass it explicitly, but with bind, any further arguments are automatically forwarded.
Let’s start with an example to learn in detail :
Step-1: First we will write a simple event handling code
2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
import React ,{Component} from "react"; import ReactDOM from "react-dom"; class Employee extends Component{ state ={ name: "Pradeep", id:1 }; // event handler with arrow function handleClick=()=>{ console.log("Button Click"); }; render(){ return( <> <h1>Hello Employee</h1> <h1>Hello, {this.state.name}</h1> <button onClick={this.handleClick}>Click Me</button> </> ) } } ReactDOM.render(<Employee />, document.getElementById("root")); |
In the above example, i created an Employee class component. Here you can see, i also define state without constructor, because i am not passing any props with component. handleClick is an event handler with arrow function which is calling from onClick button. Right now we are not passing any argument using event handler.
Step-2: Now we will pass an argument but under the Event Handler using another function or method
2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 |
import React ,{Component} from "react"; import ReactDOM from "react-dom"; class Employee extends Component{ state ={ name: "Pradeep", id:1 }; // getting argument here handleClick=(id)=>{ console.log(id); }; // passing argument under event handler with another method or function handleClickArg=()=>{ this.handleClick(this.state.id); } render(){ return( <> <h1>Hello Employee</h1> <h1>Hello, {this.state.name}</h1> <button onClick={this.handleClickArg}>Delete</button> </> ) } } ReactDOM.render(<Employee />, document.getElementById("root")); |
In the above example, we are passing id as argument under handleClickArg Event Handler. handleClick is an another function with is calling under Event Handler with argument.
Step-3: Now, I will pass the second argument “e” (react event object) in the above example
2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
import React ,{Component} from "react"; import ReactDOM from "react-dom"; class Employee extends Component{ state ={ name: "Pradeep", id:1 }; // getting argument here handleClick=(id,e)=>{ console.log(id); console.log(e); }; // passing second argument "e"(React event object) under event handler with another method or function handleClickArg=(e)=>{ this.handleClick(this.state.id, e); } render(){ return( <> <h1>Hello Employee</h1> <h1>Hello, {this.state.name}</h1> <button onClick={this.handleClickArg}>Delete</button> </> ) } } ReactDOM.render(<Employee />, document.getElementById("root")); |
In the above example, I am passing the react event object (e) as the second argument which is used to perform various events. When you will run above code then you will get the event objects in the console.
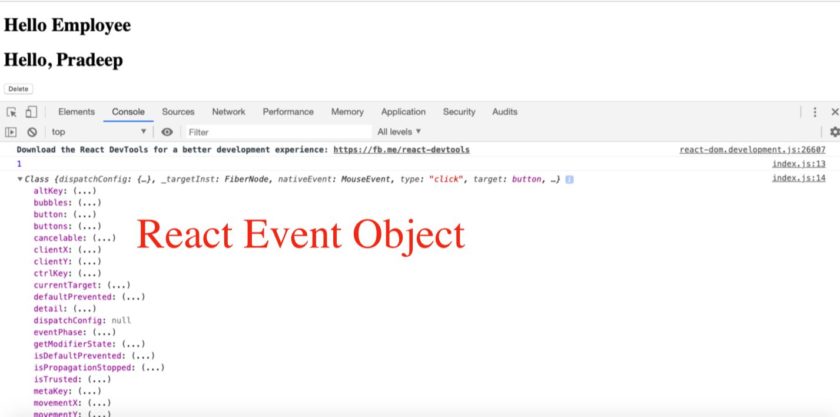
Step-4: Now, we will pass both arguments to event handler Using Arrow Function
From the above code, we will change
2 3 4 5 6 |
handleClickArg=(e)=>{ this.handleClick(this.state.id, e); } |
to (e)=>{ this.handleClick(this.state.id,e); } as anonymous arrow function and it will trigger from onClick event.
2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 |
import React ,{Component} from "react"; import ReactDOM from "react-dom"; class Employee extends Component{ state ={ name: "Pradeep", id:1 }; // Event handler with arrow function handleClick=(id,e)=>{ console.log(id); console.log(e); }; render(){ return( <> <h1>Hello Employee</h1> <h1>Hello, {this.state.name}</h1> // passing arguments using arrow function <button onClick={(e)=>{ this.handleClick(this.state.id,e); }}> Delete </button> </> ) } } ReactDOM.render(<Employee />, document.getElementById("root")); |
In the above example code, (e)=>{ this.handleClick(this.state.id,e); } is an anonymous arrow function which is calling from onClick as event handler.
Passing arguments to event handler Using Bind Function or Method
2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 |
import React ,{Component} from "react"; import ReactDOM from "react-dom"; class Employee extends Component{ state ={ name: "Pradeep", id:1 }; // Event handler with arrow function handleClick=(id,e)=>{ console.log(id); console.log(e); }; render(){ return( <> <h1>Hello Employee</h1> <h1>Hello, {this.state.name}</h1> // passing arguments using bind function <button onClick={this.handleClick.bind(this, this.state.id)}> Delete </button> </> ) } } ReactDOM.render(<Employee />, document.getElementById("root")); |
In the above code, I only need to replace
2 3 4 |
<button onClick={(e)=>{ this.handleClick(this.state.id,e); }}> Delete </button> |
With the below code:
2 3 4 |
<button onClick={this.handleClick.bind(this, this.state.id)}> Delete </button> |
You don’t need to pass “e” (React Event Object) as an argument. With the bind method React Event Object automatically calls under event handler.
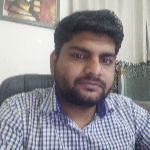
Pradeep Maurya is the Professional Web Developer & Designer and the Founder of “Tutorials website”. He lives in Delhi and loves to be a self-dependent person. As an owner, he is trying his best to improve this platform day by day. His passion, dedication and quick decision making ability to stand apart from others. He’s an avid blogger and writes on the publications like Dzone, e27.co