What is Event?
Events are the activities to which javaScript can respond. For example:
- Clicking an element
- Submitting a form
- Scrolling page
- Hovering an element
Handling Events:
The handling of React events is very similar to the handling of DOM events. There are some syntactic differences:
- React events are named using camelCase, rather than lowercase.
- With JSX you pass a function as the event handler, rather than a string.
In HTML
2 3 4 |
<button onclick="clickMe()">Click Me</button> |
Event In React:
Event with Function Component:
2 3 4 |
<button onClick={clickMe}>ClickMe</button> |
Class Component:
2 3 4 |
<button onClick={this.clickMe}>ClickMe</button> |
Note:- onClick is a camelCase Naming Convention in React. In HTML we have to use clickMe() but In React we have to use only clickMe or this.clickMe. Not need to use () with clickMe in React.
You can’t return false to prevent default behavior to React. You must call preventDefault explicitly.
Prevent default behavior in HTML:
2 3 4 |
<a href="#" onclick="console.log('Clicked Successfully'); return false"> Click Me</a> |
In the above example, you can return false to prevent default behavior with onclick event.
Prevent default behavior In React:
2 3 4 5 6 7 8 9 |
function handleClick(e){ e.preventDefault(); console.log('Clicked'); } <a href="#" onClick="{handleClick}">Click Me</a> |
In the above example, you can not return false to prevent the default behavior. For this, you need to pass “e” (synthetic event) as an argument in the function and write e.preventDefault() before any statement in React.
When using React you should generally not need to call addEventListener
to add listeners to a DOM element after it is created. Instead, just provide a listener when the element is initially rendered.
Event Handler with Class Component
2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 |
import React, {Component} from 'react'; import ReactDOM from 'react-dom'; class Employee extends Component{ constructor(props){ super(props); this.state={ name: this.props.name, count:this.props.count, }; this.clickme=this.clickme.bind(this); } clickme() { let count=this.state.count; this.setState({count: ++count}); } render(){ return ( <> <h1>Hello Employee.</h1> <h1>Name: {this.state.name}</h1> <h1>Total Click: {this.state.count}</h1> <button onClick={this.clickme}>Click Me</button> </> ) } } ReactDOM.render(<Employee name="Pradeep Maurya" count="0"/>,document.getElementById("root")); |
In the above example, When you will use class component then you need to bind “this” event handler in the constructor to access state value in the “clickme” event.
2 3 4 |
this.clickme=this.clickme.bind(this); |
if you will not bind “this” object then it will show an error in clickme event that state is not defined.
If you don’t want to bind this event handler in the constructor then, you can use an arrow function in the callback.
For Example:
2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 |
import React, {Component} from 'react'; import ReactDOM from 'react-dom'; class Employee extends Component{ constructor(props){ super(props); this.state={ name: this.props.name, count:this.props.count, }; } clickme=()=> { let count=this.state.count; this.setState({count: ++count}); } render(){ return ( <> <h1>Hello Employee.</h1> <h1>Name: {this.state.name}</h1> <h1>Total Click: {this.state.count}</h1> <button onClick={this.clickme}>Click Me</button> </> ) } } ReactDOM.render(<Employee name="Pradeep Maurya" count="0"/>,document.getElementById("root")); |
In the above example, you can see an arrow function in-class component. An Arrow function automatically bind this.
2 3 4 5 6 7 8 |
clickme=()=>{ let count=this.state.count; this.setState({count: ++count}); } |
If you will use Above arrow function instead of the normal function, then you don’t need to bind this like this.clickme=this.clickme.bind(this) in construction.
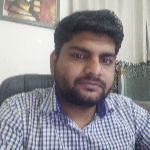
Pradeep Maurya is the Professional Web Developer & Designer and the Founder of “Tutorials website”. He lives in Delhi and loves to be a self-dependent person. As an owner, he is trying his best to improve this platform day by day. His passion, dedication and quick decision making ability to stand apart from others. He’s an avid blogger and writes on the publications like Dzone, e27.co