Updating is the process of changing state or props of component and update changes to nodes already in DOM.
An update can be caused by changes to props or state. These methods are called in the following order when a component is being re-render:
- constructor(props)
- static getDerivedStateFromProps()
- shouldComponentUpdate()
- render()
- getSnapshotBeforeUpdate()
- componentDidUpdate()
Constructor():-
The constructor for a React component is called before it is mounted. It is a special function that will get called whenever a new component is created. super(props) Directly overwrite this.state.
React constructor are only used for two purposes:
– initializing state
Ex: this.state ={name:”Tutorials website”}
– Binding the event handlers
Ex:- this.handleClick= this.handleClick.bind(this);
getDerivedStateFromProps(props,state):-
getDerivedStateFromProps is invoked right before calling the render method. When the state of the component depends on changes in props over time, Set the state. This method doesn’t have access to the component instance.
2 3 4 5 6 7 |
static getDerivedStateFromProps(prop,state){ console.log("static keyword ie required before getDrivedStateFromProps"); return null; } |
shouldComponentUpdate():
use shouldComponentUpdate() to let React know if a component’s output is not affected by the current change in state or props, It means should React re-render or it can skip rendering?
shouldComponentUpdate() is invoked before rendering when new props or states are being received. This method returns true by default. render() will not be invoked if shouldComponentUpdate return false.
Syntax:
2 3 4 5 6 7 8 |
shouldComponentUpdate(nextProps, nextState){ console.log("shouldComponentUpdate is invoked"); return true; } |
render():-
The render() method is the only required method in a class component.
- Only the required method
- Read props & state and return JSX
- Children component lifecycle methods are also executed.
getSnapshotBeforeUpdate():
This method is called right before the virtual DOM is about to make a change to the DOM (before DOM is updated), which allows our components to capture the current value or some information from the DOM before it is potentially changed. Any value returned by this lifecycle will be passed as the third parameter to componentDidUpdate().
Syntax:
2 3 4 5 6 7 |
getSnapshotBeforeUpdate(prevProps, prevState){ console.log("getSnapshotBeforeUpdate is invoked"); } |
componentDidUpdate():
componentDidUpdate() is invoked immediately after updating occurs.This method is not called for the initial render.
This method is used to re-trigger the third-party libraries used to make sure these libraries also update and reload themselves.
componentDidUpdate() will not be invoked if shouldComponentUpdate() returns false.
if your component implements the getSnapshotBeforeUpdate() lifecycle (which is rare), the value it return will be passed as a third “snapshot” parameter to componentDidUpdate(). Otherwise, this parameter will be undefined.
Syntax:-
2 3 4 5 6 7 |
componentDidUpdate(prevProps, prevState, snapshot){ console.log("componentDidUpdate is invoked"); console.log(snapshot); } |
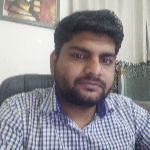
Pradeep Maurya is the Professional Web Developer & Designer and the Founder of “Tutorials website”. He lives in Delhi and loves to be a self-dependent person. As an owner, he is trying his best to improve this platform day by day. His passion, dedication and quick decision making ability to stand apart from others. He’s an avid blogger and writes on the publications like Dzone, e27.co