Mounting is the process of creating an element and inserting it in a DOM tree.
Following methods are called in following order when an instance of a component is being created and inserted into the DOM:-
- Constructor()
- getDerivedStateFromProps()
- render()
- componentDidMount()
Constructor():-
The constructor for a React component is called before it is mounted. It is a special function that will get called whenever a new component is created. super(props) Directly overwrite this.state.
React constructor are only used for two purposes:
– initializing state
Ex: this.state ={name:”Tutorials website”}
– Binding the event handlers
Ex:- this.handleClick= this.handleClick.bind(this);
getDerivedStateFromProps(props,state):-
getDerivedStateFromProps is invoked right before calling the render method. When the state of the component depends on changes in props over time, Set the state. This method doesn’t have access to the component instance.
2 3 4 5 6 7 |
static getDerivedStateFromProps(prop,state){ console.log("static keyword ie required before getDrivedStateFromProps"); return null; } |
render():-
The render() method is the only required method in a class component.
- Only the required method
- Read props & state and return JSX
- Children component lifecycle methods are also executed.
componentDidMount():-
componentDidMount() is invoked immediately after a component is mounted. This method is executed once in a lifecycle of a component and after the first render() method.
- This is the best place to perform any ajax calls to load data.
- The API calls should be made in the componentDidMount method always.
Let’s create an example to understand the mounting lifecycle method
2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 |
import React ,{Component} from "react"; import ReactDOM from "react-dom"; class Employee extends Component{ constructor(props){ super(props); this.state={ count:0 } console.log("Constructor"); } incrementCount=()=>{ this.setState({ count: this.state.count +1, }); } static getDerivedStateFromProps(prop,state){ console.log("static keyword ie required before getDrivedStateFromProps"); return null; } componentDidMount(){ console.log("Component Did Mount"); } render(){ console.log("Rendering"); return ( <> <h1>Hello Employee</h1> <h1>count: {this.state.count}</h1> <button onClick={this.incrementCount}>Increment</button> </> ); } } ReactDOM.render(<Employee/>, document.getElementById("root")); |
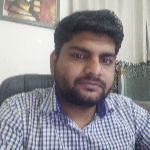
Pradeep Maurya is the Professional Web Developer & Designer and the Founder of “Tutorials website”. He lives in Delhi and loves to be a self-dependent person. As an owner, he is trying his best to improve this platform day by day. His passion, dedication and quick decision making ability to stand apart from others. He’s an avid blogger and writes on the publications like Dzone, e27.co