In this article, we will learn about how to use an image with HTML file and JSX component using “img” tag in Reactjs. Adding images with the JSX component in react js is an important part of any designer or developer. If you will see the React App default directory structure then you will get two option to upload your images file:
- Inside public Folder
- Inside src Folder
Inside public Folder
If you put a file into the public folder, it will not be processed by Webpack. Instead, it will be copied into the build folder untouched.
To reference asset in the public folder, you need to use a special variable called “PUBLIC_URL”. You can only access the image with %PUBLIC_URL% prefix, from the public folder.
Usually, I recommend importing stylesheet, images, and fonts from javascript.
2 3 4 5 |
<link rel="favicon icon" href="%PUBLIC_URL%/favicon.ico"/> <link rel="stylesheet" type="text/css" href="%PUBLIC_URL%/styles.css"/> |
Note:
- None of the files in the public folder get post-processed or minified.
- Missing files will not be called at compilation time, and will cause 404 errors for your users.
- Result filenames won’t include content hashes so you will need to add query arguments or rename them every time they change.
Using Public Folder
- You need a file with a specific name in the build output, such as manifest.webmanifest.
- You have thousands of images and need to dynamically reference their paths.
- You want to include a small script like custom.js outside of the bundled code.
- Some libraries may be incompatible with Webpack and you have no other option but to include it as a <script> tag.
For Example: if your image is in Public Folder
index.html
2 3 4 5 6 7 8 |
<img src="%PUBLIC_URL%/mypic.jpg" alt="mypic"/> //if your image is in another folder under the public folder like: public/images then <img src="%PUBLIC_URL%/images/mypic.jpg" alt="mypic"/> |
App.js
For the javascript module, you need to use {process.env.PUBLIC_URL} instead of %PUBLIC_URL%.
2 3 4 5 |
<img src={process.env.PUBLIC_URL + "/mypic.jpg"} alt="mypic"/> <img src={process.env.PUBLIC_URL + "/images/mypic.jpg"} alt="mypic"/> |
Inside src Folder
With Webpack, using static assets like images and fonts works similar to CSS. You can import a file right in a JavaScript module. This tells Webpack to include that file in the bundle. Unlike CSS imports, importing a file gives you a string value. This value is the final path you can reference in your code. For example: As the src attribute of an image or the href of a link to a PDF.
Note:
- Script and stylesheet get minified and bundled together to avoid extra network requests.
- Missing files cause compilation errors instead of 404 errors for your users.
- Result filenames include content hashes so you don’t need to worry about browsers caching their old versions.
How to use
This ensures that when the project is built, Webpack will correctly move the images into the build folder, and provide us with correct paths.
App.js
2 3 4 5 |
import pic from './mypic.jpg'; <img src={pic} alt="mypic"/> |
Example Code:
2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
import React, {Component} from "react"; import ReactDOM from "react-dom"; import logo from "./tutorials-website-logo.png"; class Employee extends Component{ render(){ return( <> <h2>Inside Public Folder</h2> <img src={process.env.PUBLIC_URL+"tutorials-website-logo.png"} alt="tutorialswebsite logo"/> <h2>Inside src Folder</h2> <img src={logo} alt="tutorialswebsite logo"/> </> ); } } ReactDOM.render(<Employee/>, document.getElementById("root")); |
output:
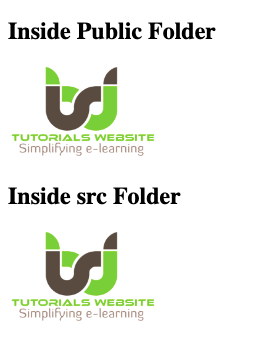
Inspect element code:
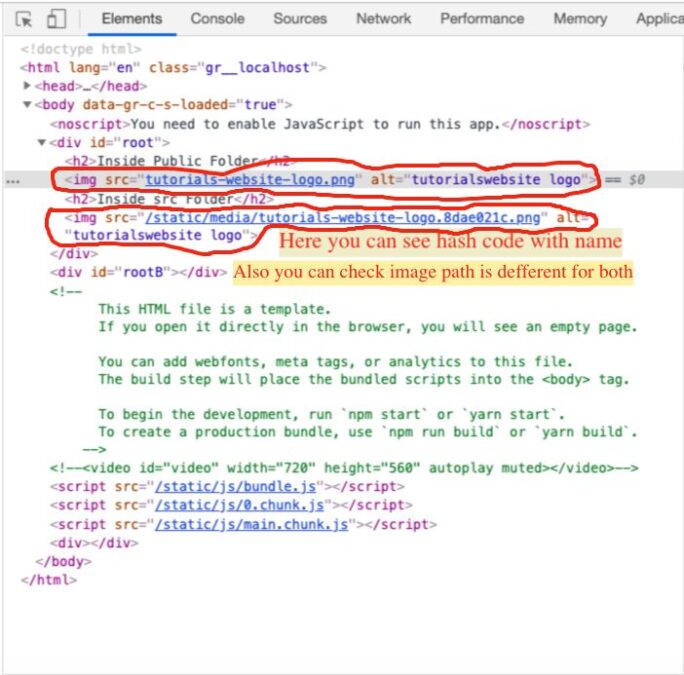
Conclusion:
If you are creating an e-commerce website where you need to upload a lot’s images then I will recommend you to use “Public” Folder. You can’t use the src folder at this time due to security issues.
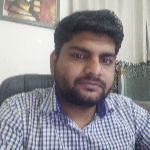
Pradeep Maurya is the Professional Web Developer & Designer and the Founder of “Tutorials website”. He lives in Delhi and loves to be a self-dependent person. As an owner, he is trying his best to improve this platform day by day. His passion, dedication and quick decision making ability to stand apart from others. He’s an avid blogger and writes on the publications like Dzone, e27.co